I have been living in Japan for nearly 4 years, but this year is truly special to me. The most important point is that I finally knew exactly what to study. During high school, mathematics was my world, was the one I loved. However, I had been lost after high school’s graduation about my major. There were too many choices while I had too little knowledge to decide.
Fortunately, everything has happened to me in 2012 helps me make up my mind. Now I know Machine Learning, Artificial Intelligence and related fields are the ones for me for the rest of my life.
After high school, I directly got into Hanoi University of Technology (HUT). Since I wanted to learn about robotics, I chose Automatic Control with the hope of learning about controlling real robots. However, I soon found out that my department in general, and my major in particular had very little thing to do with robot. I was baffled at first, but then that problem became unimportant as I would have a chance to choose my major again when coming to Japan. I am happy that I made a right decision this time, even though the outcome is not what I expected.
I spent the first year in Japan at Japanese Language Center for International Students with other MEXT’s fellowships. Not until December 2009, were my major and university decided. Therefore, I took a lot of advices from my Sempai by listening to their experiences and preferences. I still had an affection for robotics, so I opted for Mechano-Informatics which would satisfy my quest for robotics’ study. Up until now, I can say that that was the right decision, but I could never predict what I have become now. My study now is hardly related to robot’s control theory, instead, I focus on Artificial Intelligence and Machine Learning, which involve lots of probabilistic mathematics and algorithms’ design.
The funniest thing was that I chose Mechano-Informatics not purely because I was interested in robotics but also because I was told that my study would require a tiny amount of programming. I seriously hated programming and anything related to IT at that time. This sounds strange, because many people assume that people being good at maths would automatically are good at programming. That should have been true for me, but because I did not pay enough attention to my first programming class (first semester at HUT), I became afraid of it. That hatred feeling lasted until last summer vacation when I spend two months in Vietnam. I would have never spent time studying during homecoming trip, but that was a special occasion. As I mentioned above, I had an programming class in Vietnam before, which adversely made me hate programming. However, after knowing that there would be numerous programming classes in my department’s curriculum, I got worried. My original intention was very plain, I planned to study fundamental algorithms and programming concepts during two months in Vietnam in order to “survive” those classes. I was that scared!!! Before coming back to Japan, I finished the most well-known Vietnamese programming book (the only Vietnamese one I have read) for beginners written by Quack Tuan Ngoc. It was helpful, and I thought I was quite ready for class in the following semester. My caution turned out to be excessive, since the Software I class was very good class for beginners. It was quite easy, even if the previous vacation had not existed. My interest toward programming had been growing over time, especially after I started play with micro controllers.
I was suddenly attracted by low level programming for micro controllers in two months, last November and December. Even though it was fleeting, it did play an important role in luring me to the world of programming and algorithms. I soon gave up low-level programming as the result of taking Software II class by professor Hitoshi Iba. The Software I class of my department was good, but it was basically about practicing programming, which could not satisfy a maths “nerd” like me. Consequently, I sought another programming class from Electronics-Informatics department. The class started from the middle for the semester, when I already had a quite solid background in programming. Prof Hitoshi’s teaching method was great in my opinion. Software II was totally different from Software I, the later taught me programming technique while the former taught me algorithms. Programming only is boring, but with the companion of algorithms’ design, it becomes great. I mastered all fundamental abstract data structures (ADTs) and C language after finishing the final report. Thankful to Software II class, I got rid of low-level programming and totally switched to high-level design. I am taking Artificial Intelligence class by professor Hitoshi Iba (will take another class of him next semester). This class sums up my programming and algorithms’ study. I spend about 10 hours per week on average on weekly reports, from essential graph search to genetic programming. It does make me tired, but it never bored me. The feeling of 10 continuous hours for maths has come back to me. I am at my best when I study with passion.
My story about my growing affection for programming ends here. From this April, the time I have spent for algorithms’ study is on par with the time I spent for mathematics during high school. Reading algorithms’ book has become my hobby now, which certainly will make me a better programmer. I believe by the time of graduation, my confidence in programming will be far stronger.

Programming and Algorithms alone obviously could not make 2012 such as special year to me. The other one is my current and future study focus, which are Artificial Intelligence and Machine Learning. My affection for those fields of study is naturally ignited by my first Neural Network’s implementation. When I first heard about Neural Network, I thought it was cool from the name to the implementation. I had no theoretical knowledge about Neural Network at that time, so I quickly read about it and grasp its core ideas. I followed several tutorials to make back propagation neural network as well as self organizing map. Implementation neural network was really, really fun.
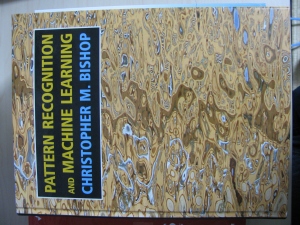
The event that played the most important role in pushing me closer to ML and AI was the summer internship at Microsoft. It was so far the best summer of my life. Details about my internship can be found here:
http://www.bing.com/community/site_blogs/b/jp/archive/2012/12/06/2012-msd-2-l.aspx
The bottom line is that I have seen how important and fascinating Machine Learning is during my internship. Additionally, I am taking 4 classes that related to AI and ML this semester, which makes them even more appealing to me. I have planned to make Machine Learning the theme for my thesis next year, and I am really looking forward to it. When I was thirteen, Maths found me, and I am now very happy to meet another inspirational subject, Machine Learning.